- Home
- Flutter 101
- BLoC in Flutter
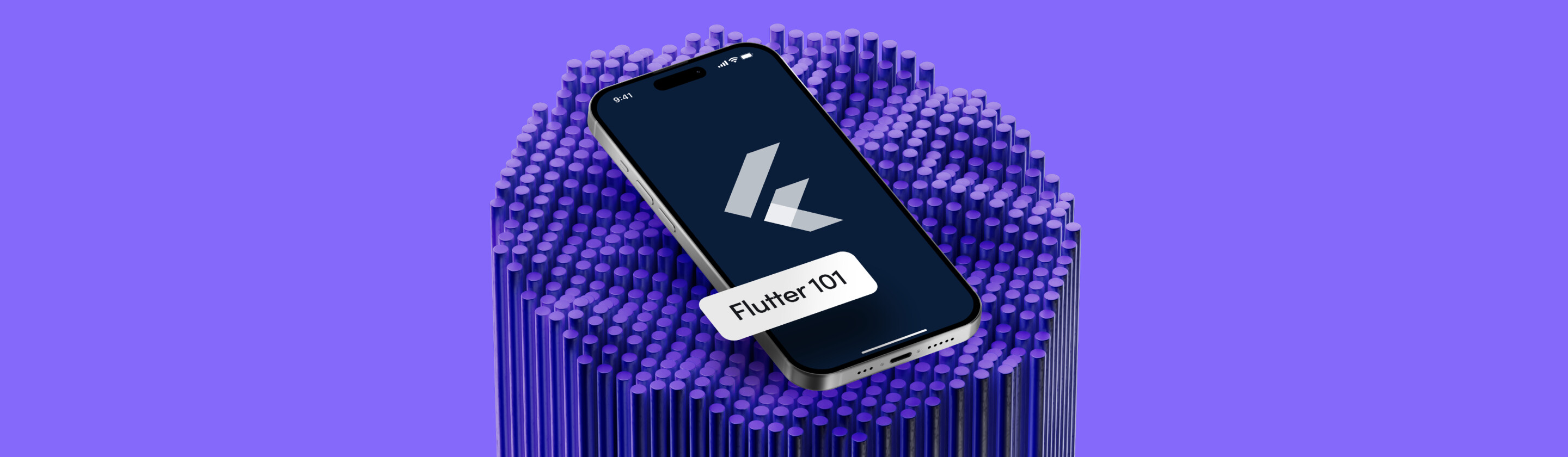
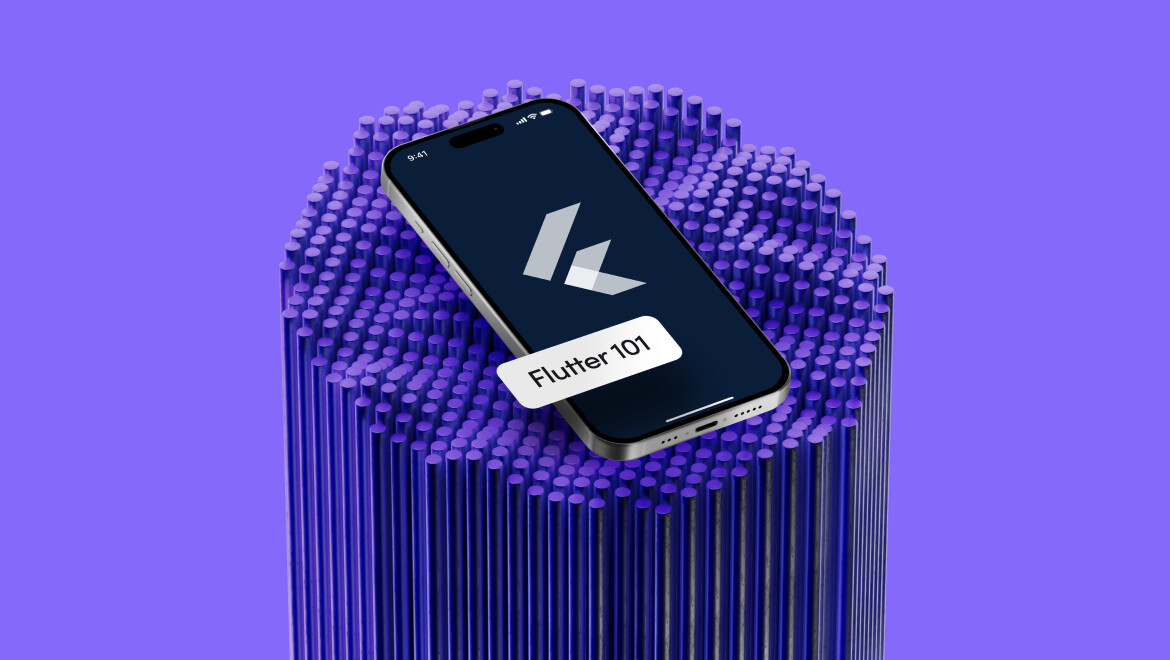
BLoC in Flutter
BLoC in Flutter
What is BLoC in Flutter?
BLoC (Business Logic Component) is a design pattern in Flutter that helps manage state and business logic in a structured and reusable way. By separating the business logic from the UI, BLoC enables cleaner code, better testability, and a more scalable app architecture. BLoC is part of the reactive programming paradigm, which means it uses Streams to handle events and states, making it easier to manage complex state changes in Flutter applications.
Core Concepts of BLoC in Flutter:
- Business Logic Layer: In the BLoC pattern, the business logic (such as API calls, data processing, etc.) is decoupled from the UI code. The BLoC handles all the logic and sends only the necessary states back to the UI for rendering.
- Streams and Sinks: BLoC leverages Dart’s Streams for managing the flow of data. The Sink is used to add events or inputs into the stream, while the Stream itself is used to listen for output states.
- Events and States:
- Event: Represents user actions or external triggers (like a button press).
- State: Represents the current condition or data that the UI needs to render.
How BLoC Works in Flutter:
- Event Input: The UI sends events (e.g., button clicks) to the BLoC.
- Processing Logic: The BLoC processes the input events, handles business logic, such as fetching data from an API or database, and manipulates data accordingly.
- State Output: The processed state is sent back to the UI, which listens to the BLoC’s state stream and updates the view accordingly.
Benefits of Using BLoC in Flutter:
- Separation of Concerns: BLoC clearly separates the business logic from the UI, making the code more modular and maintainable.
- Scalability: It provides a scalable way to manage complex state changes across large apps, making it easier to add new features without breaking existing code.
- Reusability: The BLoC pattern encourages reusability, as the business logic can be reused across multiple parts of the app or even different apps.
- Testability: Since the logic is separated from the UI, testing becomes easier. You can write unit tests for the BLoC without needing to test the UI.
Practical Example:
BLoC is ideal for apps that require complex state management or asynchronous data handling, such as real-time chat apps, financial apps, or news apps. For instance, in a shopping app, BLoC can handle the product catalog, user authentication, and cart management, ensuring that the UI displays the correct data in real time.
In summary, BLoC in Flutter is a powerful pattern for managing state and business logic, providing a clean and scalable solution for building robust Flutter applications.
Ready to discover more terms?