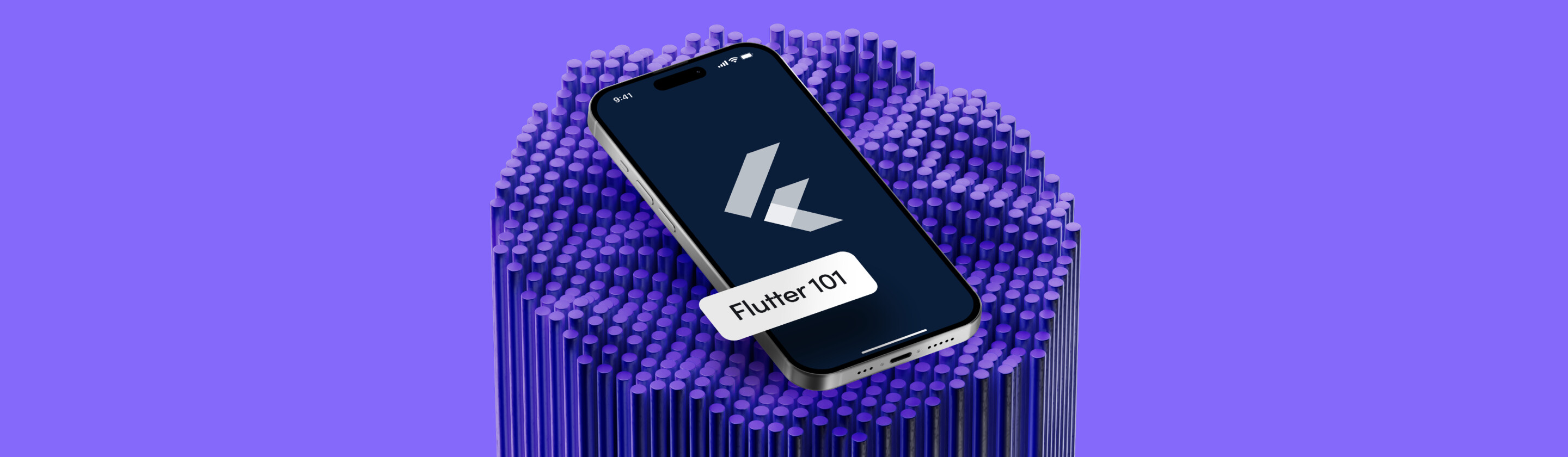
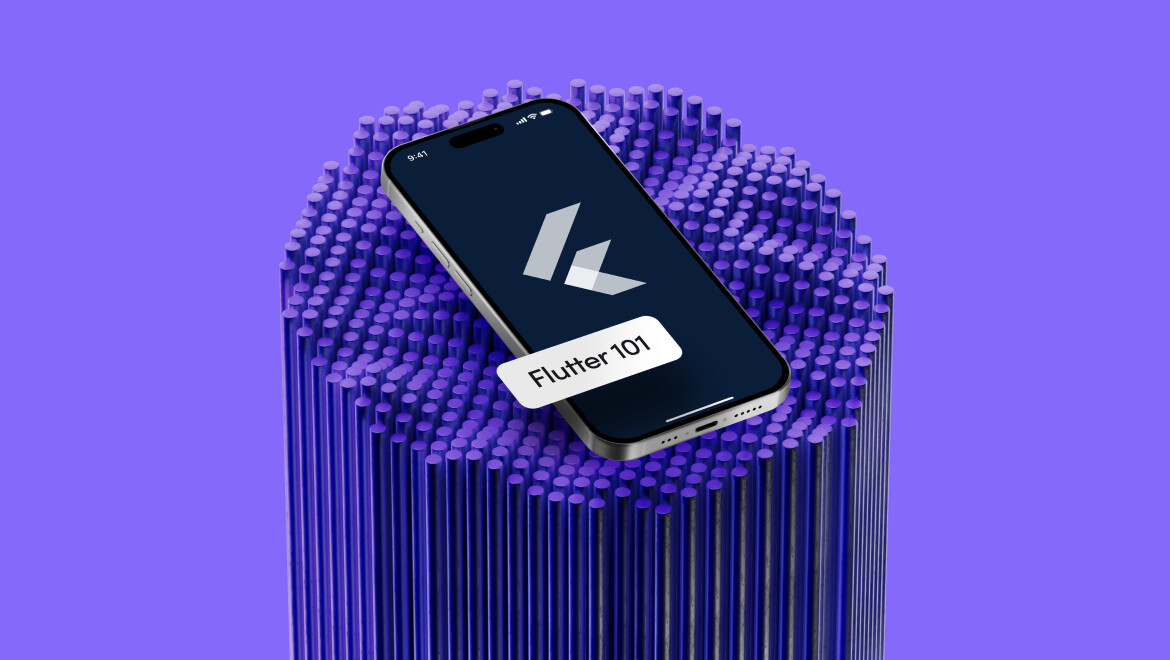
State Management in Flutter
What is Flutter State Management?
In Flutter, state management is the process of controlling how an app’s data (or state) changes and updates the user interface (UI). Effective state management ensures that an app behaves responsively and efficiently when the underlying data changes. Since Flutter applications are built using widgets, each widget can have its own state, and managing this efficiently becomes crucial, especially as apps grow in complexity.
Core Concepts in Flutter State Management
- State: In Flutter, state is the data that influences a widget’s behavior or appearance. For instance, in a counter app, the number displayed on the screen represents the app’s state.
- Stateless vs Stateful Widgets:
- Stateless Widgets: These widgets are static and don’t update once built. They are used for displaying static content, such as text or icons.
- Stateful Widgets: These can change their state during runtime, like a button that increments a counter when clicked.
- Rebuilding the UI: Flutter uses a reactive UI model, meaning that when a widget’s state changes, it automatically rebuilds the affected parts of the UI to reflect the new state.
Popular Flutter State Management Techniques
- setState():
- What it is: The most basic method of state management in Flutter. It updates the widget’s state and triggers a rebuild whenever data changes.
- Use case: Perfect for small, simple apps where state is localized to a few widgets.
- Provider:
- What it is: Provider is a popular state management solution in Flutter that wraps around InheritedWidget, simplifying sharing and managing state across an app.
- Use case: Ideal for apps that require state to be shared across multiple widgets and for projects that need a more scalable approach. Provider is widely used in enterprise app development due to its efficiency and simplicity. If you’re interested in how Flutter supports large-scale apps, you can learn more about its benefits for enterprise app development.
- BLoC (Business Logic Component):
- What it is: BLoC separates business logic from the UI by using Streams. It’s a reactive approach that handles complex state changes by mapping user inputs (events) to different app states.
- Use case: Excellent for larger apps with complex, asynchronous data flows, such as real-time updates or apps that rely heavily on API calls. BLoC is frequently used in cross-platform development, where managing state across different platforms efficiently is key.
- GetX:
- What it is: GetX is a lightweight, reactive state management solution that combines state management, dependency injection, and routing into one easy-to-use package.
- Use case: Preferred for projects that require minimal setup and fast, reactive changes without much boilerplate code.
Why is State Management Crucial?
Efficient state management ensures that only the necessary parts of the app rebuild when state changes, improving performance. It also helps maintain a clean separation between the UI and the business logic, which makes code easier to maintain and test, especially in large applications.
If you’re curious about real-world applications, several successful apps have been built using Flutter’s state management solutions. For example, you can explore the Topline music recording app, which demonstrates how Flutter’s efficient state management helped streamline its development.
In conclusion, selecting the right state management technique in Flutter depends on your app’s complexity. Whether you use basic setState(), Provider, or a more advanced solution like BLoC, Flutter provides flexible and scalable options to meet various app development needs.
Ready to discover more terms?